The whole number portion is the minute part of min: sec per mile D. Multiply the decimal portion of this number x 60 E. The decimal portion x 60 is the seconds part of min: sec per mile Example: Find the pace of a runner who is running at 7.8 mph. 1 / 7.8 = 0.1282 B. 0.1282 x 60 = 7.692 C. The minutes part of pace is 7 D. Computing MIN in an array with large elements We usually assign a high value to MIN to compute minimum value in an array. But if an array has large elements, we must assign the highest possible value to the array. Below is the C implementation. C/C Code Generation Generate C and C code using MATLAB® Coder™. Usage notes and limitations: If you specify an empty array for the second argument in order to supply dim or nanflag, the second argument must be of fixed-size and of dimension 0 -by- 0.
C++ Algorithm min() function can be used in following 3 ways:
- It compares the two values passed in its arguments and returns the smaller between them, and if both are equal, then it returns the first one.
- It also compares the two values using a binary function, which is defined by the user, and then passed as argument in std::min().
- It is also used to find the smallest element in a given list, and it returns the first one if there are more than one are smallest in the list.
Elements are compared using operator< for the first version or using the given binary comparison function comp for the second version.
Syntax
Parameter
a: first value to compare.
C++ Maximum Function
b: Second value to compare.
comp: A user defined binary predicate function that accepts two arguments and returns true if the two arguments are in order and false otherwise. It follows the strict weak ordering to order the elements.
il: An initializer_list with the values to compare.
Return value
It returns the smaller of a and b. If the values are equivalent, returns a.
Returns the smallest value in il. If several values are equivalent to the minimum, returns the left most such value.
Complexity
Complexity is linear in one less than the number of elements compared.
Exceptions
This function throws an exception if any comparison throws an exception.
Please note that invalid parameters cause an undefined behavior.
Example 1
Let's see the simple example to demonstrate the use of min():
Output:
Example 2
Let's see another simple example to demonstrate the use of min() using default version:
Min In C++
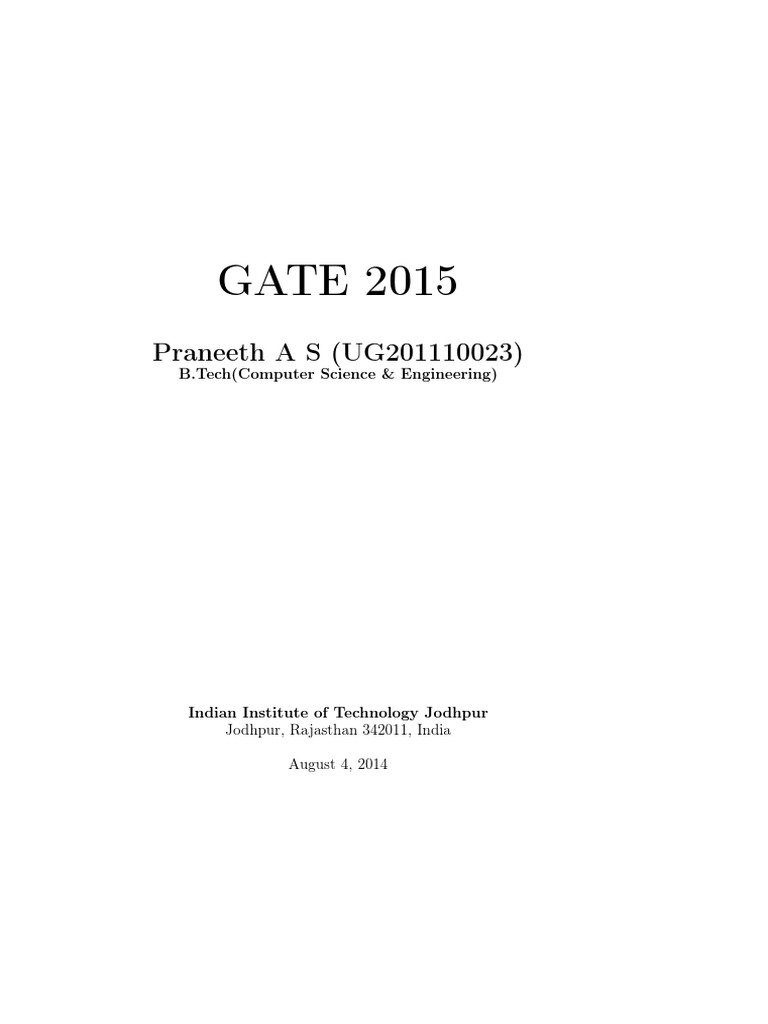
Output:
Example 3
Let's see another simple example to demonstrate the use of min() using predicate function comp:
Output:
Example 4
Let's see a simple example to find the minimum element in the list:

Output:
A binary heap is a heap data structure created using a binary tree.
binary tree has two rules –
- Binary Heap has to be a complete binary tree at all levels except the last level. This is called a shape property.
- All nodes are either greater than equal to (Max-Heap) or less than equal to (Min-Heap) to each of its child nodes. This is called heap property.
Implementation:
- Use an array to store the data.
- Start storing from index 1, not 0.
- For any given node at position i:
- Its Left Child is at [2*i] if available.
- Its Right Child is at [2*i+1] if available.
- Its Parent Node is at [i/2]if available.
Heap Majorly has 3 operations –
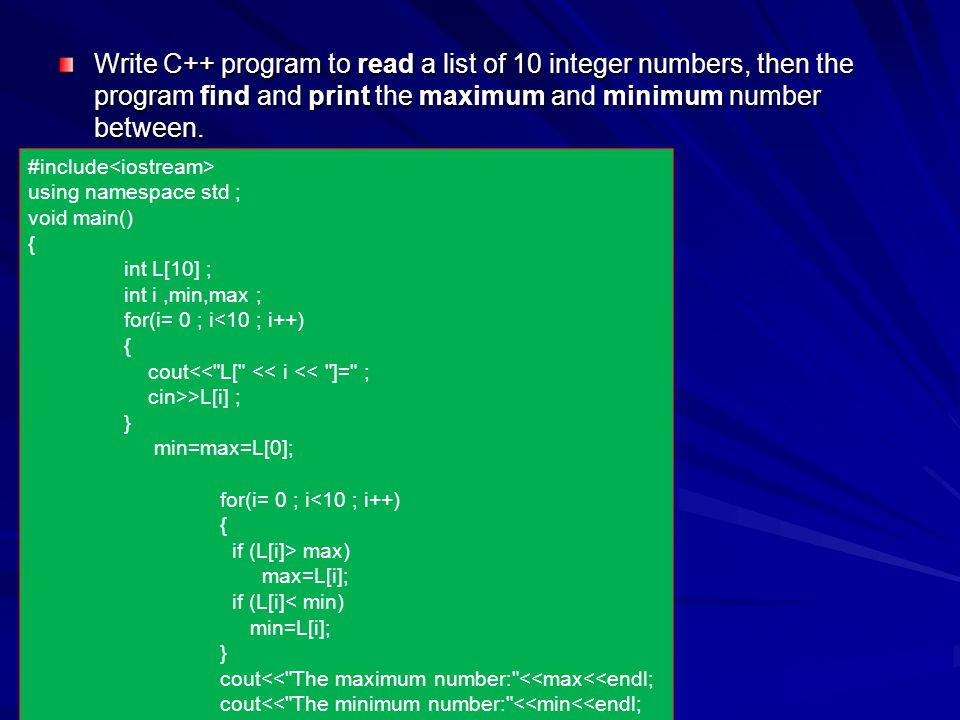
- Insert Operation
- Delete Operation
- Extract-Min (OR Extract-Max)
Insert Operation:
- Add the element at the bottom leaf of the Heap.
- Perform the Bubble-Up operation.
- All Insert Operations must perform the bubble-up operation(it is also called as up-heap, percolate-up, sift-up, trickle-up, heapify-up, or cascade-up)
Extract-Min OR Extract-Max Operation:
- Take out the element from the root.( it will be minimum in case of Min-Heap and maximum in case of Max-Heap).
- Take out the last element from the last level from the heap and replace the root with the element.
- Perform Sink-Down
- All delete operations must perform Sink-Down Operation ( also known as bubble-down, percolate-down, sift-down, trickle-down, heapify-down, cascade-down).
Sink-Down Operation:
- If the replaced element is greater than any of its child node in case of Min-Heap OR smaller than any if its child node in case of Max-Heap, swap the element with its smallest child(Min-Heap) or with its greatest child(Max-Heap).
- Keep repeating the above step, if the node reaches its correct position, STOP.
Delete Operation:
- Find the index for the element to be deleted.
- Take out the last element from the last level from the heap and replace the index with this element .
- Perform Sink-Down
Time and Space Complexity:
Complete Code for Min-Heap:
How To Find Max And Min In C++
Java
Min In C++ Vector
Output:
Related Posts:
