Angular for JavaScript; Cheat Sheet (v 2.X.Y) TypeScript. Powered by Google ©2010-2017. Code licensed under an MIT-style License. Documentation licensed under CC BY 4.0. This cheat sheet co-authored by Ravi Kiran and Suprotim Agarwal, aims at providing a quick reference to the most commonly used features in AngularJS. It will also make you quickly productive with Angular. This article is from the Free DNC Magazine for.Net and JavaScript developers. Abstract: This Angular 10 Cheatsheet tutorial details significant changes in Angular 9 and some new features introduced in Angular 10. It also contains info to upgrade to Angular 10. Angular is a popular framework for JavaScript/ TypeScript application development.
Updated June 6, 2018
AngularJS Cheat Sheet Review. Review of AngularJS Cheat Sheet powered by the Slant community. Ranked in these Questions Question Ranking #7.
In this post I give an overview of the most uses Angular CLI command.
Using the CLI commands can greatly boost your productivity on Angular projects.
Create a new app
Create a new angular application with the default styling option CSS.
Create new angular project with scss styling and a routing module.
More info: https://github.com/angular/angular-cli/wiki/new
Build and start the app
Build the app and start it as a webserver.
You can reach the app at: http://localhost:4200/
Build your app and open it in the webbrowser
Serve your app on a different port and use ssl.
Your app will be here: https://localhost:4444/
Build using Ahead of Time compilation.
This is useful to test aot, which will be used when you compile for production.
Serve your app inside a base url
This will run the app below the url /my-app.
Your app will be here: http://localhost:4200/my-app/
This is useful when you are deploying later to a similar URL and want to develop with this URL too.
More info on ng serve : https://github.com/angular/angular-cli/wiki/serve
Build the app
Default build
Compiles the application into an output directory.
The build artifacts will be stored in the dist/ directory.
Angularjs Cheat Sheet For Beginners
Production build
Productie build for a app with a base url: /my-app/
Environment test Build for a app with a base url: /my-app/
Build dev environment with all production elements enabled
Provide an output path for the compiled code.
Useful when automating your workflow.
More info on build: https://github.com/angular/angular-cli/wiki/build

Update the app
Updates the current application to latest versions.
See the update without making changes.
Run through without making any changes.
Will list all files that would have been created when running ng update.
More info: https://github.com/angular/angular-cli/wiki/update
Generate
Generates the specified blueprint.
You can also use the shorter notation: ng g
I will use the short notations in the examples below.
For the full notation see the official documentation.
Generate a module
Generate a module with a routing module

This is handy when creating a lazy loading module.
Generate a component
Generate a component without a spec file
Generate a interface
Generate an interface with a “type”
This will create an interface with the file name: my-interface.green.ts.
Generate a class
Generate an enumeration
Generate a service
Generate a guard
Generate a pipe
Generate a directive
Simulating a generate command.
Add the option –dry-run (-d) to run generate without making any changes.
It will list all files that would have been created when running ng generate.
More information on generate: https://github.com/angular/angular-cli/wiki/generate
Best practises.
For each feature of your app create a module and create your other components inside this module.
This will make it easy to eager or lazy load this feature of your app.
See also Angular style guid: https://angular.io/guide/styleguide#feature-modules
Example cars feature
- Create a module cars with the routing module

- Next create components inside this module
Angularjs Cheat Sheet 2020
Create interfaces, classes and services inside a shared folder of this module.
- Create a interface (model) for the cars in a shared folder inside this module
- Create a service to fetch data in the shared folder inside this module.
This results in the following directory structure
Read more about the Angular CLI: https://github.com/angular/angular-cli/wiki
Learn more about Angular’s best practices on the official Angular style guide: https://angular.io/guide/styleguide
Create vs. Get AngularJS application
The module dependency array tells AngularJS to create an app, otherwise to load it:
Create myApp | var app = angular.module('myApp', []); |
Get myApp | var app = angular.module('myApp'); |
Binding
@
Text binding, expressions are supported using{{ }}
=
Two-way binding&
Method binding<
One-way binding (usually for components)?
for an optional binding e.g.@?
for an optional string binding
Service, Factory, Provider, Value & Controller
- A
Service
is a singleton instance of a custom object. In can be used to share state or usually to wrap the remote REST API in a reuseable faction. It is injected using the name (review sample).
Basically simple AngularJs beans we want to inject later.
- The
Factory
follows as the name already tells the factory pattern and so allows us to construct either a complexvalue
we want to inject into our controllers or even already existing JS objects which require any dependencies upfront. Basically wrap beans which need a constructor injection (review sample).
Provider
- A
Provider
is the base forfactory
andservice
and has the biggest flexibility. Most important it can be injected into the config method and so configured before the first usage (review sample).
- A
Value
is a simple object, int or string we want to make available for injection
HTML elements in AngularJS
Access HTML element Directive
The easiest way is just to use the link method:
Access HTML element in Component
In a component we can just inject the $element
.
Access raw HTML element
As you can see in the last example we can access the raw DOM element using [0]
on the wrapped element.
Restrict in Angular Directive
The restrict
option is typically set to:
'A'
– only matches attribute name'E'
– only matches element name'C'
– only matches class name'M'
– only matches comment
These restrictions can all be combined as needed:
'AEC'
– matches either attribute or element or class name
URL Routing to AngularJS components
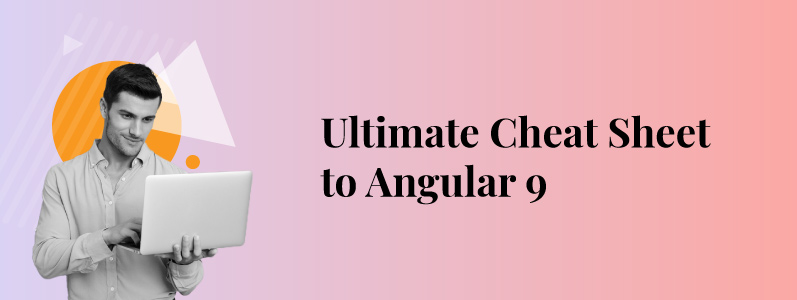
ocLazyLoad angular components during routing
As the lifecycle of components is a bit different we have to eager load them using ocLazyLoad:
Access URL parameters
The easiest way to access the URL params is just to inject $stateParams
into the component get read it. A bit cleaner is using the resolve
of the state
to inject the parameters into the component. As so we have to keep in mind that happens async, which means we have to implement the $onInit
method to get hold of the injected values into our component. More to components.
Dynamic ncyBreadcrumb label for AngularJS Components
Accessing just the $scope
doesn’t always work well during the reload of the page furthermore, it doesn’t work for components anyhow. The easiest way is just to inject the $breadcrumb
service and be done with it:
